Modbus API¶
Module: typhoon.api.modbus
Modbus Client Initialization¶
To initialize one TCP based Modbus client first you should make object of TCPModbusClient()
class
Many of Modbus client options can be set directly in constructor or later by using dedicated functions:
import typhoon.api.modbus as modbus
# create instance of the Modbus TCP client
# in case any arguments are invalid ValueError exception will be raised
try:
modbus_client = modbus.TCPModbusClient(host="192.168.0.250",
port=502,
auto_open=True)
except ValueError:
print("Invalid arguments...")
Create the Modbus client and set options with the dedicated functions:
import typhoon.api.modbus as modbus
# create instance of the Modbus TCP client
# in case any arguments are invalid ValueError exception will be raised
try:
modbus_client = modbus.TCPModbusClient()
except ValueError:
print("Invalid arguments...")
# set host
modbus_client.set_host("192.168.0.250")
# set port
modbus_client.set_port(502)
# enable auto open feature
modbus_client.set_auto_open(True)
Nearly all Modbus API functions raise one or more exceptions in order to distinguish different errors that can occur.
Modbus exceptions hierarchy are displayed below.
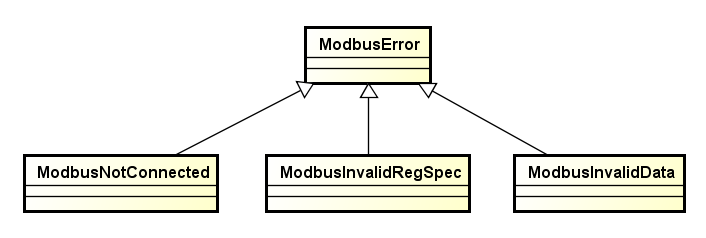
If you want to catch all Modbus exceptions you should catch base ModbusError
exception:
from typhoon.api.modbus.exceptions import ModbusError
try:
modbus_client.read_input_registers_adv("501i,[502,503]u,[504,505,506,507]f")
except ModbusError as ex:
print(ex)
In case you want to distinguish different errors you should catch them separately:
from typhoon.api.modbus.exceptions import ModbusError, ModbusNotConnected, ModbusInvalidRegSpec
try:
modbus_client.read_input_registers_adv("501i,[502,503]u,[504,505,506,507]f)
except ModbusNotConnected as ex:
print(ex)
# doo something in case connection is not opened
except ModbusInvalidRegSpec as ex:
print(ex)
# doo something in case provided registers specification is invalid
except ModbusError as ex:
print(ex)
# doo something in case read error occurs
API references¶
- class TCPModbusClient(host='localhost', port=502, unit_id=1, timeout=30.0, debug=False, auto_open=True, auto_close=False)¶
Class that implements functionalities of the TCP based Modbus client.
The class itself is the proxy to ModbusClent() class of the PyModbusTCP library (http://pythonhosted.org/pyModbusTCP/package/class_ModbusClient.html) with a additional functionalities.
- __init__(host='localhost', port=502, unit_id=1, timeout=30.0, debug=False, auto_open=True, auto_close=False)¶
Creates a instance of the TCPModbusClient. Client parameters can be set here or later by using appropriate functions (
set_host()
,set_port()
etc)- Parameters:
host (str) – hostname or IPv4/IPv6 address server address (optional)
port (int) – TCP port number (optional)
unit_id (int) – unit ID (optional)
timeout (float) – socket timeout in seconds (optional)
debug (bool) – debug state (optional)
auto_open (bool) – auto TCP connect on first request (optional)
auto_close (bool) – auto TCP close after each request (optional)
- Returns:
TCPModbusClient object
- Return type:
- Raises:
ValueError – if input parameters’ values are incorrect
- modbus()¶
Return wrapped ModbusClent object.
- Returns:
ModbusClent object
- Return type:
ModbusClent
- endianness()¶
Returns currently set endianness :return:
- set_endianness(endian_type)¶
Sets endianness that will be used for merging multiple 16 bit registers’ values in one 32 bit or 64 bit number
- Parameters:
endian_type –
little_endian
orbig_endian
or appropriate constants can be used:
TCPModbusClient.BIG_ENDIAN
TCPModbusClient.LITTLE_ENDIAN
- Raises:
ModbusError – in case endianness type is not supported
- auto_close()¶
Returns status of auto TCP close mode. :returns: auto close mode status (
True
activated orFalse
deactivated) :rtype: bool
- set_auto_close(state)¶
Set auto TCP close mode. If this mode is active, connection will be closed after each request.
- Parameters:
state (bool) – Activate/deactivate auto close mode
- auto_open()¶
Returns status of auto TCP connect mode. :returns: auto open mode status (
True
activated orFalse
deactivated) :rtype: bool
- set_auto_open(state)¶
Set auto TCP connect mode. If this mode is active, connection will be opened on the first request.
- Parameters:
state (bool) – Activate/deactivate auto open mode
- close()¶
Closes TCP connection.
- Returns:
close status (
True
if connection successfully closed orNone
if connection already closed)- Return type:
bool or None
- open()¶
Connect to modbus server (open TCP connection)
- Returns:
connect status (
True
if connection successfully opened otherwise returnFalse
)- Return type:
bool
- debug()¶
Returns status of debug mode.
- Returns:
debug mode status (
True
activated orFalse
deactivated)- Return type:
bool
- set_debug(state)¶
Set debug mode.
Note
While debug mode is active, debug information will be writen to the console.
- Parameters:
state (bool) – Activate/deactivate debug mode
- host()¶
Returns current host.
- Returns:
hostname
- Return type:
str
- set_host(hostname)¶
Set host (IPv4/IPv6 address or hostname like ‘plc.domain.net’)
- Parameters:
hostname (str) – hostname or IPv4/IPv6 address
- Raises:
ModbusError – if hostname is invalid or cannot be set
- port()¶
Returns current TCP port.
- Returns:
TCP port value
- Return type:
int
- set_port(port)¶
Set TCP port.
- Parameters:
port (int) – TCP port number
- Raises:
ModbusError – if port is invalid or cannot be set
- timeout()¶
Returns current timeout.
- Returns:
socket timeout value
- Return type:
float
- set_timeout(timeout=None)¶
Set socket timeout.
- Parameters:
timeout (float) – socket timeout in seconds (0 to 3600)
- Raises:
ModbusError – if timeout is invalid or cannot be set
- unit_id()¶
Returns current unit ID.
- Returns:
unit ID value
- Return type:
int
- set_unit_id(unit_id=None)¶
Sets unit ID field.
- Parameters:
unit_id (int) – unit ID (0 to 255)
- Raises:
ModbusError – if unit ID is invalid or cannot be set
- is_open()¶
Get status of TCP connection.
- Returns:
status (
True
if connection is opened otherwise returnFalse
)- Return type:
bool
- read_coils(bit_addr, bit_nb=1, to_int=False)¶
Implementation of Modbus function READ_COILS (0x01).
Reads
bit_nb
number of successive addresses starting frombit_addr
address.- Parameters:
bit_addr (int) – bit address (0 to 65535)
bit_nb (int) – number of bits to read (1 to 2000)
to_int – convert coils values to integer number
Note
Value read from
bit_addr
address is treated as MSB- Returns:
list of booleans (
True
orFalse
) or integer number ifto_int==True
- Return type:
list of bool
- Raises:
ModbusError (base exception) – if error occurs during read of coils
ModbusNotConnected – if connection is not opened before this function is called
- read_discrete_inputs(bit_addr, bit_nb=1, to_int=False)¶
Implementation of Modbus function READ_DISCRETE_INPUTS (0x02)
Reads
bit_nb
number of successive addresses starting frombit_addr
address.- Parameters:
bit_addr (int) – bit address (0 to 65535)
bit_nb (int) – number of bits to read (1 to 2000)
to_int – convert coils values to integer number
Note
Value read from
bit_addr
address is treated as MSB- Returns:
list of integers (
1
or0
) or integer number ifto_int==True
- Return type:
list of bool
- Raises:
ModbusError (base exception) – if error occurs during read of discrete inputs
ModbusNotConnected – if connection is not opened before this function is called
- read_input_registers(reg_addr, reg_nb=1)¶
Implementation of Modbus function READ_INPUT_REGISTERS (0x04)
- Parameters:
reg_addr (int) – register address (0 to 65535)
reg_nb (int) – number of registers to read (1 to 125)
- Returns:
registers list
- Return type:
list of int
- Raises:
ModbusError (base exception) – if error occurs during read of input registers
ModbusNotConnected – if connection is not opened before this function is called
- read_holding_registers(reg_addr, reg_nb=1)¶
Implementation of Modbus function READ_HOLDING_REGISTERS (0x03)
- Parameters:
reg_addr (int) – register address (0 to 65535)
reg_nb (int) – number of registers to read (1 to 125)
- Returns:
registers list
- Return type:
list of int
- Raises:
ModbusError (base exception) – if error occurs during read of holding registers
ModbusNotConnected – if connection is not opened before this function is called
- read_input_registers_adv(read_spec)¶
Advance function for reading input registers.
- Parameters:
read_spec – string with registers specification
Note
To specify registers specification special simple language is used. More about
Registers Specification Language
you can read here.- Returns:
data list with values converted to specified registers types
- Return type:
list of unsigned/signed int or float numbers depending read specification
- Raises:
ModbusError (base exception) – if error occurs during read of input registers
ModbusNotConnected – if connection is not opened before this function is called
ModbusInvalidRegSpec – if registers specification (
read_spec
) is not correct
- read_holding_registers_adv(read_spec)¶
Advance function for reading holding registers.
- Parameters:
read_spec – string with registers specification
Note
To specify registers specification special simple language is used. More about
Registers Specification Language
you can read here.- Returns:
data list with values converted to specified registers types
- Return type:
list of unsigned/signed int or float numbers depending read specification
- Raises:
ModbusError (base exception) – if error occurs during read of input registers
ModbusNotConnected – if connection is not opened before this function is called
ModbusInvalidRegSpec – if registers specification (
read_spec
) is not correct
- write_single_coil(bit_addr, bit_value)¶
Implementation of Modbus function WRITE_SINGLE_COIL (0x05)
Write
bit_value
value onbit_addr
address.- Parameters:
bit_addr (int) – bit address (0 to 65535)
bit_value (bool) – bit value to write
- Raises:
ModbusError (base exception) – if error occurs during write of single coil
ModbusNotConnected – if connection is not opened before this function is called
- write_single_register(reg_addr, reg_value)¶
Implementation of Modbus function WRITE_SINGLE_REGISTER (0x06)
Write
reg_value
value onreg_addr
address.- Parameters:
reg_addr (int) – register address (0 to 65535)
reg_value (int) – register value to write
- Raises:
ModbusError (base exception) – if error occurs during write of single register
ModbusNotConnected – if connection is not opened before this function is called
- write_multiple_coils(bits_addr, bits_value)¶
Implementation of Modbus function WRITE_MULTIPLE_COILS (0x0F)
Write
bits_value
values starting frombits_addr
address.- Parameters:
bits_addr (int) – bits address (0 to 65535)
bits_value (list) – bits values to write
- Raises:
ModbusError (base exception) – if error occurs during write of multiple coils
ModbusNotConnected – if connection is not opened before this function is called
- write_multiple_registers(regs_addr, regs_value)¶
Implementation of Modbus function WRITE_MULTIPLE_REGISTERS (0x10)
Write
regs_value
values starting fromregs_addr
address.- Parameters:
regs_addr (int) – registers address (0 to 65535)
regs_value (list) – registers values to write
- Raises:
ModbusError (base exception) – if error occurs during write of multiple registers
ModbusNotConnected – if connection is not opened before this function is called
- write_registers_adv(write_spec, regs_values)¶
Advance function for writing multiple registers.
- Parameters:
write_spec – string with registers specification
Note
To specify registers specification special simple language is used. More about
Registers Specification Language
you can read here.- Parameters:
regs_values (list of unsigned/signed int or float numbers depending write specification) – registers values to write.
- Raises:
ModbusError (base exception) – if error occurs during write of multiple registers
ModbusNotConnected – if connection is not opened before this function is called
ModbusInvalidRegSpec – if registers specification (
write_spec
) is not correctModbusInvalidData – if provided registers values are not compatible with registers types
Utility module¶
- bool_list_to_int(bool_list)¶
Converts given bool list to the int number. First element in given bool list is used as MSB.
- Parameters:
bool_list (list) – List of booleans.
- Returns:
An integer.
- uint16_to_int16(uint16, big_endian=True)¶
Convert unsigned int (16 bit) number to signed int (16 bit) number.
- Parameters:
uint16 (int) – unsigned int (16 bit) number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
singed int (16 bit) number
- Return type:
int
- uint32_to_int32(uint32, big_endian=True)¶
Convert unsigned int (32 bit) number to signed int (32 bit) number.
- Parameters:
uint32 (int) – unsigned int (32 bit) number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
singed int (32 bit) number
- Return type:
int
- uint64_to_int64(uint64, big_endian=True)¶
Convert unsigned int (64 bit) number to signed int (64 bit) number.
- Parameters:
uint64 (int) – unsigned int (64 bit) number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
singed int (64 bit) number
- Return type:
int
- uint32_to_float(uint32, big_endian=True)¶
Convert unsigned int (32 bit) number to float number.
- Parameters:
uint32 (int) – unsigned int (32 bit) number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
float number
- Return type:
float
- uint64_to_double(uint64, big_endian=True)¶
Convert unsigned int (64 bit) number to float number (64 bit).
- Parameters:
uint64 (int) – unsigned int (64 bit) number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
float number (64 bit)
- Return type:
float
- float_to_uint32(float_num, big_endian=True)¶
Convert float number to unsigned int (32 bit) number.
- Parameters:
float_num (float) – float number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
int number
- Return type:
int
- double_to_uint64(double_num, big_endian=True)¶
Convert float number (64 bit) to unsigned int (64 bit) number.
- Parameters:
double_num (float) – float number (64 bit)
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
int number
- Return type:
int
- int16_to_uint16(int16, big_endian=True)¶
Convert signed int (16 bit) number to unsigned int (16 bit) number.
- Parameters:
int16 (int) – signed int (16 bit) number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
unsigned int (16 bit) number
- Return type:
int
- int32_to_uint32(int32, big_endian=True)¶
Convert signed int (32 bit) number to unsigned int (32 bit) number.
- Parameters:
int32 (int) – signed int (32 bit) number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
unsigned int (32 bit) number
- Return type:
int
- int64_to_uint64(int64, big_endian=True)¶
Convert signed int (64 bit) number to unsigned int (64 bit) number.
- Parameters:
int64 (int) – signed int (64 bit) number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
unsigned int (64 bit) number
- Return type:
int
- int32_to_uint16_list(int32, big_endian=True)¶
Converts signed int number (32 bit) to list of two unsigned int (16 bit) numbers.
- Parameters:
int32 (int) – signed int number (32 bit)
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
list with two unsigned int (16 bit) values
- Return type:
list
- int64_to_uint16_list(int64, big_endian=True)¶
Converts signed int number (64 bit) to list of four unsigned int (16 bit) numbers.
- Parameters:
int64 (int) – signed int number (64 bit)
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
list with four unsigned int (16 bit) values
- Return type:
list
- uint32_to_uint16_list(uint32, big_endian=True)¶
Converts unsigned int number (32 bit) to list of two unsigned int (16 bit) numbers.
- Parameters:
uint32 (int) – unsigned int number (32 bit)
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
list with two unsigned int (16 bit) values
- Return type:
list
- uint64_to_uint16_list(uint64, big_endian=True)¶
Converts unsigned int number (64 bit) to list of four unsigned int (16 bit) numbers.
- Parameters:
uint64 (int) – unsigned int number (64 bit)
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
list with four unsigned int (16 bit) values
- Return type:
list
- float_to_uint16_list(float_num, big_endian=True)¶
Converts float number to list of two unsigned int (16 bit) numbers.
- Parameters:
float_num (float) – float number
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
list with two unsigned int (16 bit) values
- Return type:
list
- double_to_uint16_list(double_num, big_endian=True)¶
Converts float number (64 bit) to list of four unsigned int (16 bit) numbers.
- Parameters:
double_num (float) – float number (64 bit)
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
list with four unsigned int (16 bit) values
- Return type:
list
- uint16_list_to_float(int_list, big_endian=True)¶
Converts list of two unsigned int (16 bit) numbers to float number.
- Parameters:
int_list (list) – list with two unsigned int (16 bit) values
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
float number
- Return type:
float
- uint16_list_to_double(int_list, big_endian=True)¶
Converts list of four unsigned int (16 bit) numbers to float number (64 bit).
- Parameters:
int_list (list) – list with four unsigned int (16 bit) values
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
float number (64 bit)
- Return type:
float
- uint16_list_to_int32(int_list, big_endian=True)¶
Converts list of two unsigned int (16 bit) numbers to signed int (32 bit).
- Parameters:
int_list (list) – list with two unsigned int (16 bit) values
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
signed int number (32 bit)
- Return type:
int
- uint16_list_to_int64(int_list, big_endian=True)¶
Converts list of four unsigned int (16 bit) numbers to signed int number (64 bit).
- Parameters:
int_list (list) – list with four unsigned int (16 bit) values
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
signed int number (64 bit)
- Return type:
int
- uint16_list_to_uint32(int_list, big_endian=True)¶
Converts list of two unsigned int (16 bit) numbers to unsigned int (32 bit) number.
- Parameters:
int_list (list) – list with two unsigned int (16 bit) values
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
unsigned int (32 bit)
- Return type:
int
- Raise:
ValueError: in case
int_list
length is != 2
- uint16_list_to_uint64(int_list, big_endian=True)¶
Converts list of four unsigned int (16 bit) numbers to unsigned int (64 bit) number.
- Parameters:
int_list (list) – list with four unsigned int (16 bit) values
big_endian (bool) – True for big endian/False for little (optional)
- Returns:
unsigned int (64 bit)
- Return type:
int
- Raise:
ValueError: in case
val_list
length is != 4